Signing up through an email address is always going to be a difficult user story but this is made especially tricky when app signup is concerned. This is because there is always a point where they new user needs to leave the app to check their email. This email confirmation invariably contains a link which will, by default send the user onto the web to finish the signup flow by entering a password. With linkalist this works, as all apps have a web version, but there remains a difficulty because the user will still need to log into the app with their new password after entering it.
Android Deep Links
This is where Android App Links or Deep Links come in. With these links, clicking the link in the email will take the user back into the app. With Deep Links, the user is presented with a choice to use the app or just go back the browser. With App Links, this choice is made for the user. For now, we're limited the behaviour to just enable Deep Links as it can be somewhat difficult to set up. The main difference is that Deep Links can be done entirely on the client while App Links need some server-side work as well.
Configuring linkalist for Deep Links
<activity
android:name=".activities.DiveListActivity"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
<intent-filter android:label="Divelist Registration">
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<!-- Accepts URIs that begin with "http://www.example.com/gizmos” -->
<data
android:host="divelist.mobi"
android:pathPrefix="/index.php/nonuser/user_invite"
android:scheme="https"/>
<data
android:host="*.divelist.mobi"
android:pathPrefix="/index.php/nonuser/user_invite"
android:scheme="https"/>
</intent-filter>
</activity>
<intent-filter android:label="Divelist Password">
<action android:name="android.intent.action.VIEW"/>
<category android:name="android.intent.category.DEFAULT"/>
<category android:name="android.intent.category.BROWSABLE"/>
<data
android:host="divelist.mobi"
android:pathPrefix="/index.php/nonuser/user_change_password"
android:scheme="https"/>
<data
android:host="*.divelist.mobi"
android:pathPrefix="/index.php/nonuser/user_change_password"
android:scheme="https"/>
</intent-filter>
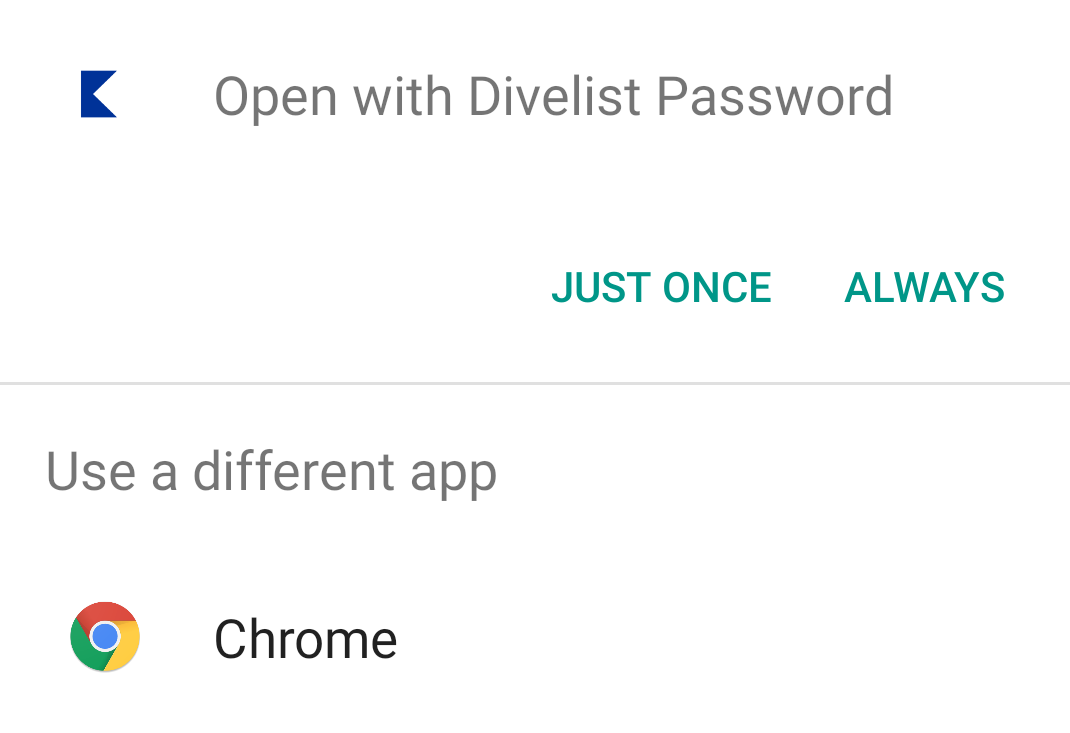
Launch Activity Changes
boolean isDeepLink = LinkalistCore.getInstance().parseDeepLinkIntent(getIntent());
if (true == isDeepLink)
{
EnterPasswordActivity.launchActivity(this);
}
else
{ .... // whatever was here before }
if ((LoginActivity.REQUEST_LOGIN == requestCode) || (EnterPasswordActivity.REQUEST_ENTER_PASSWORD == requestCode))
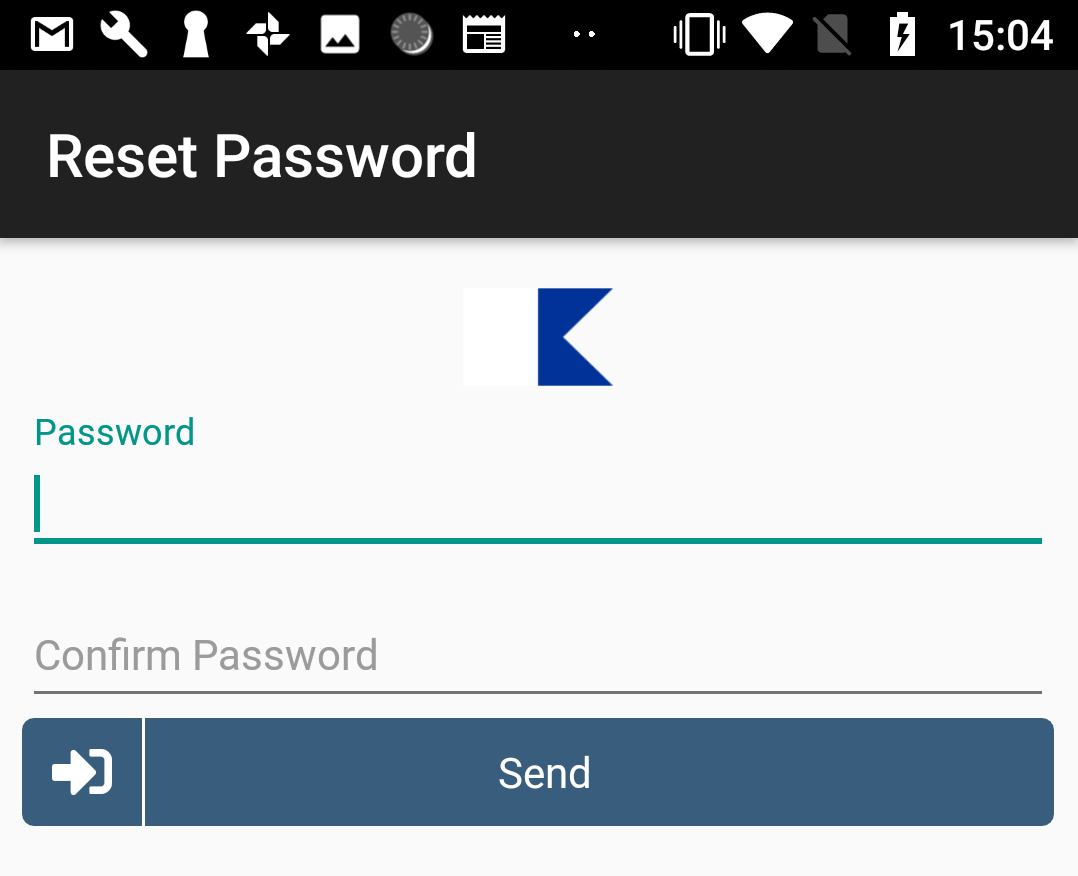
LinkalistCore.getInstance().requirePasswordConfirmation(false);
And that is basically it - a minimal effort way to do a user email signup while keeping the user in your app as much as possible.